Kotlin StateFlow vs SharedFlow: Understanding the Differences
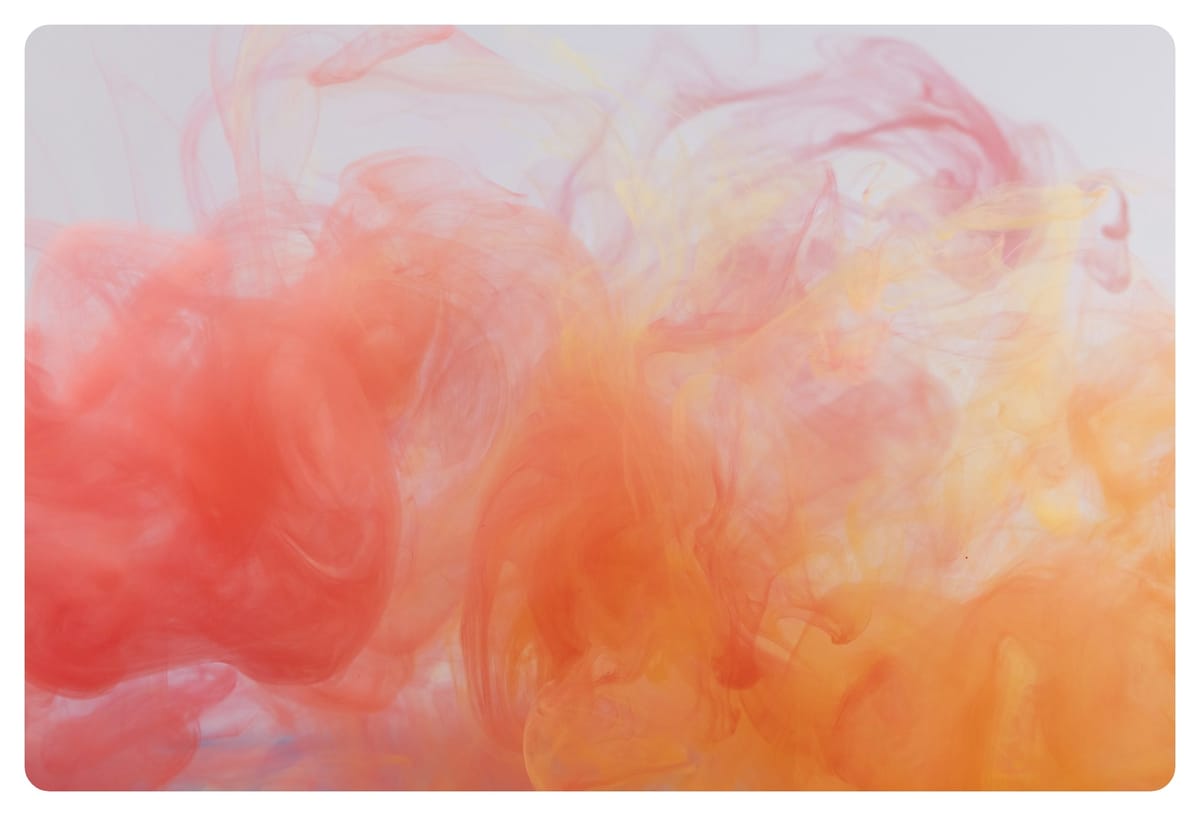
Kotlin's Flow API is a powerful tool for handling streams of data in a reactive way. Within this API, two important constructs stand out: StateFlow
and SharedFlow
. Both are designed to manage state and share data across your application, but they serve slightly different purposes and have distinct behaviors. In this post, we will explore the differences between StateFlow
and SharedFlow
, when to use each, and provide some practical examples to solidify your understanding.
What is StateFlow
?
StateFlow
is a special type of Flow that is designed to represent and manage a state in a reactive way. It’s essentially a state-holder observable that emits the current and subsequent state updates to its collectors. Here are some key characteristics:
- Hot Stream:
StateFlow
is a hot stream, meaning it always has an active state and emits the latest value to any new collectors. - State Holder: It holds the latest value and replays it to new collectors, ensuring that no collector misses the latest state.
- Simplicity:
StateFlow
is simpler to use when you have a single piece of state that you need to observe.
Example Usage of StateFlow
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.flow.StateFlow
import kotlinx.coroutines.runBlocking
class ViewModel {
private val _state = MutableStateFlow("Initial State")
val state: StateFlow<String> get() = _state
fun updateState(newState: String) {
_state.value = newState
}
}
fun main() = runBlocking {
val viewModel = ViewModel()
viewModel.state.collect { state ->
println("Current State: $state")
}
viewModel.updateState("Updated State")
}
Output:
Initial State
Updated State
In this example, StateFlow holds a string state that can be updated and observed. The collector immediately gets the current state “Initial State” and will print “Updated State” when the state is updated.
What is SharedFlow
?
SharedFlow
, on the other hand, is a more general-purpose flow that can emit values to multiple collectors simultaneously. Unlike StateFlow
, SharedFlow
does not hold any state and can be configured to have different replay behaviors, making it more flexible in various scenarios.
- Hot Stream: Like
StateFlow
,SharedFlow
is also a hot stream. - No State Holding:
SharedFlow
does not hold any state by default, but you can configure it to replay a certain number of previous emissions. - Multiple Collectors: It’s designed to handle multiple collectors with more fine-tuned control over how emissions are shared and replayed.
Example Usage of SharedFlow
import kotlinx.coroutines.flow.MutableSharedFlow
import kotlinx.coroutines.flow.SharedFlow
import kotlinx.coroutines.runBlocking
class EventManager {
private val _events = MutableSharedFlow<String>()
val events: SharedFlow<String> get() = _events
suspend fun triggerEvent(event: String) {
_events.emit(event)
}
}
fun main() = runBlocking {
val eventManager = EventManager()
eventManager.events.collect { event ->
println("Event received: $event")
}
eventManager.triggerEvent("First Event")
eventManager.triggerEvent("Second Event")
}
Output:
First Event
Second Event
In this example, SharedFlow
is used to emit events. Collectors can receive these events in real-time, and since SharedFlow
doesn’t hold state by default, only the new emissions are received.
Key Differences Between StateFlow
and SharedFlow
Feature | StateFlow | SharedFlow |
---|---|---|
State Management | Holds a state and emits the latest value | No state; emits new values as they come |
Replay Capability | Always replays the latest state to new collectors | Configurable; can replay a specified number of values |
Use Case | Single source of truth (state management) | Event streams, broadcasting, or multicasting |
Initialization | Requires an initial value | No initial value required |
When to Use StateFlow
vs SharedFlow
- Use
StateFlow
when you need to manage and observe a single piece of state that should always be available to collectors. It’s ideal for scenarios like view states in an MVI architecture where you have a single source of truth. - Use
SharedFlow
when you need to broadcast events or share data among multiple collectors without maintaining a single state. It’s perfect for event-driven architectures or situations where you don’t need to hold the last emitted value.
Converting Between StateFlow
and SharedFlow
There are scenarios where you may want to convert between StateFlow
and SharedFlow
in your Kotlin code, especially if you need to transition between state management and event-driven broadcasting. Fortunately, Kotlin provides simple ways to achieve this conversion.
Converting StateFlow
to SharedFlow
If you want to convert a StateFlow
to a SharedFlow
, it’s quite straightforward. Since StateFlow
is already a Flow
, you can collect values from the StateFlow
and emit them into a SharedFlow
.
Here's an example:
// To create a StateFlow
val stateFlow: MutableStateFlow<String> = MutableStateFlow("Initial State")
// To convert StateFlow to SharedFlow
// use import kotlinx.coroutines.flow.asSharedFlow
val sharedFlow: SharedFlow<String> = stateFlow.asSharedFlow()
// To collect from SharedFlow
sharedFlow.collect { value ->
println("SharedFlow received: $value")
}
// To emit new state
stateFlow.value = "Updated State"
In this case, the asSharedFlow()
extension function allows you to convert StateFlow
to a SharedFlow
, and the newly converted flow can then be used in situations where you want to broadcast events to multiple collectors without maintaining state.
When converting StateFlow
to SharedFlow
, keep in mind that SharedFlow
does not hold a state unless you configure a replay parameter. We can use the shareIn
extension function to define a replay value.
// Convert StateFlow to SharedFlow with replay of 1 value
val sharedFloww = stateFlow
.asSharedFlow()
.shareIn(this, replay = 1, started = SharingStarted.Eagerly)
Converting SharedFlow
to StateFlow
Converting a SharedFlow
to a StateFlow
is a bit trickier, as SharedFlow
doesn’t inherently maintain state. However, you can combine SharedFlow
with a StateFlow
to accomplish this by using the last emitted value of the SharedFlow
and then storing it in a StateFlow.
Here’s an example of how to convert a SharedFlow to StateFlow:
// Create a SharedFlow
val sharedFlow = MutableSharedFlow<String>()
// Create a StateFlow initialized with a default value
val stateFlow = MutableStateFlow("Initial State")
// Launch a coroutine to collect from SharedFlow and update StateFlow
launch {
sharedFlow.collect { value ->
stateFlow.value = value
}
}
// Emit events to SharedFlow
sharedFlow.emit("Event 1")
sharedFlow.emit("Event 2")
// Collect the latest state from StateFlow
stateFlow.collect { state ->
println("StateFlow current state: $state")
}
When converting SharedFlow
to StateFlow
, you need to handle state management manually by collecting and storing the latest value in a StateFlow
.
Conclusion
Understanding when to use StateFlow
versus SharedFlow
is crucial for effective state management and event handling in Kotlin Multiplatform projects. StateFlow
is your go-to for managing a state that needs to be observed by one or more collectors, while SharedFlow
provides flexibility in handling events and broadcasting data to multiple collectors.
By mastering these tools, you can create more robust, reactive Kotlin applications that efficiently manage state and events across multiple platforms.

Take Your Android Development to the Next Level
Let’s build something amazing together. Get in touch to explore how I can assist you in bringing your Android app ideas to life with the best practices in modern Android development.