Unit vs Nothing vs Void in Kotlin: Everything You Need to Know
Initially, these types can be a bit confusing. What’s the difference between them? When should you use one over the other? Let’s break it down!
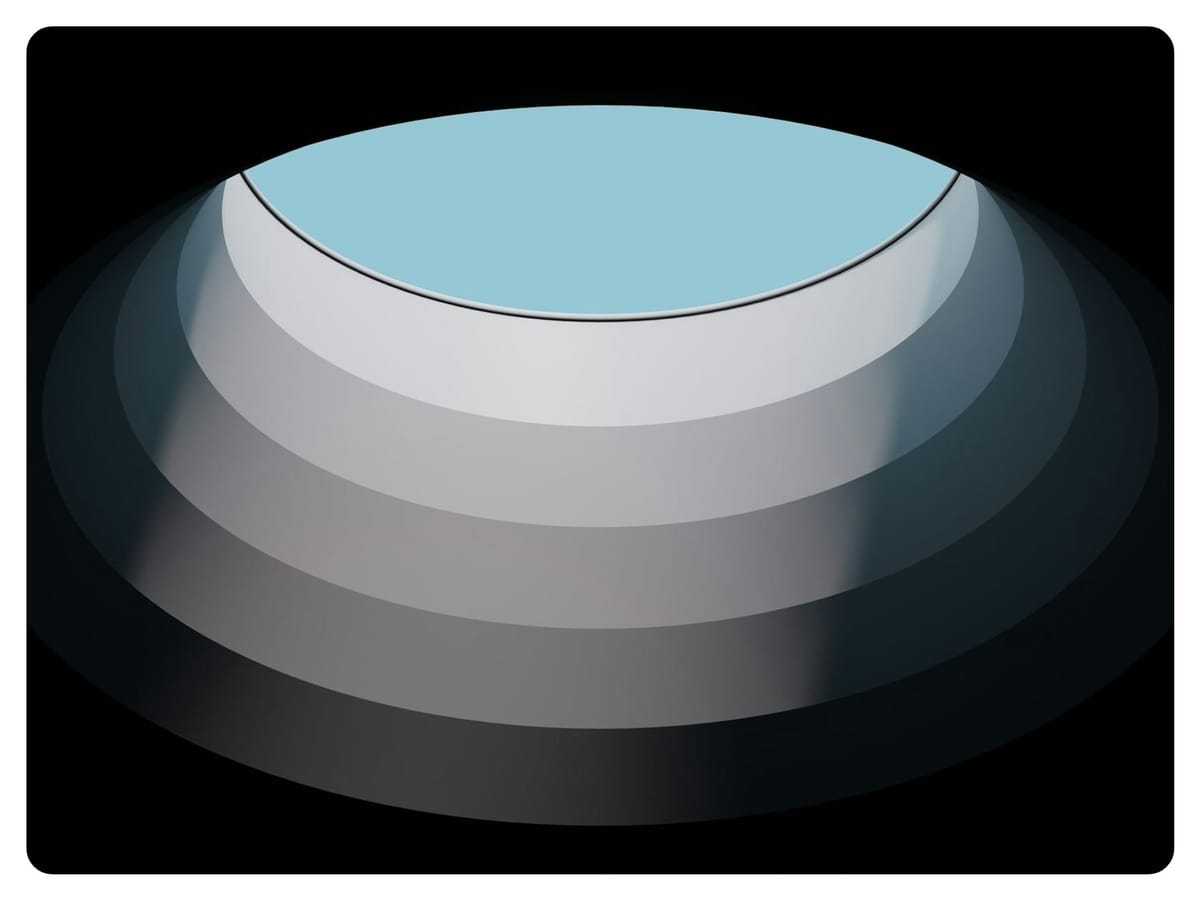
Hey there, Kotlin enthusiasts! 👋 If you’re diving into Kotlin Multiplatform (KMP) development, you’ve probably stumbled upon Unit
, Nothing
, and maybe even Void
. At first glance, these types can be a bit confusing. What’s the difference between them? When should you use one over the other? Let’s break it down!
Unit
What is Unit
In Kotlin, Unit
is a type that represents the absence of a meaningful value. If you’re coming from a Java background, you can think of it as the Kotlin equivalent of void
. But here’s the kicker: Unit
is actually a real class with a single instance.
class Unit private constructor()
Yes, that’s right! Unit has only one value—Unit. This makes it useful in functional programming paradigms where everything is an object.
When to use Unit
- as a function return type: when your function doesn’t return any meaningful value.
fun printMessage(message: String): Unit {
println(message)
}
: Unit
since it’s the default.- in Generic Parameters: when you need to specify a type but don’t have any meaningful value to return.
fun performAction(action: () -> Unit) {
action()
}
Nothing
What is Nothing
Nothing
is a special type in Kotlin that has no values. It represents a function that never returns (always throws an exception or enters an infinite loop). Nothing
is a subtype of all types, making it useful for type inference.
When to Use Nothing
- Functions that never return normally: such as functions that always throw an exception.
fun fail(message: String): Nothing {
throw IllegalStateException(message)
}
- Infinite loops: functions that loop forever without returning.
fun infiniteLoop(): Nothing {
while (true) {
// Do something endlessly
}
}
Void
What is Void
In Kotlin, Void
is not the same as void
in Java. It’s actually java.lang.Void
, and it’s mainly used for Java interoperability. Void is a reference type that has no instances.
When to use Void
- Interoperability with Java code: when you’re dealing with Java methods or libraries that use
Void
.
val task: Callable<Void> = Callable<Void> {
// Perform some action
null
}
Void
is a non-instantiable final class of Java, we can only return null
from such functions.- Reflection or Generics: In advanced scenarios where
Void
is required as a type parameter.
If you are developing a pure Kotlin project without using any Java code, you are probably not going to encounter Void
in the codebase.
Quick comparison
Type | Description | Use Case |
---|---|---|
Unit | Represents no meaningful value (has one instance) | Functions that don't return a value |
Nothing | Represents a lack of value and never returns | Functions that always throw or loop infinitely |
Void | Java's Void type (no instances) | Java interoperability and specific generic cases |
Tips and best practices
- Use
Unit
for standard functions
For most cases where you don’t need to return a value from a function, use Unit. - Leverage
Nothing
for error handling
When writing functions that always throw exceptions or don’t terminate, specifyNothing
as the return type for better clarity. - Avoid
Void
unless necessary
Unless you’re interfacing with Java code, there’s rarely a need to useVoid
in Kotlin. - Be explicit when it helps
While Kotlin allows you to omit return types in many cases, being explicit can improve code readability, especially in public APIs.
Wrapping up
Understanding the differences between Unit
, Nothing
, and Void
can help you write clearer, more idiomatic Kotlin code.
Was this guide helpful? Share it with your fellow Kotlin developers!